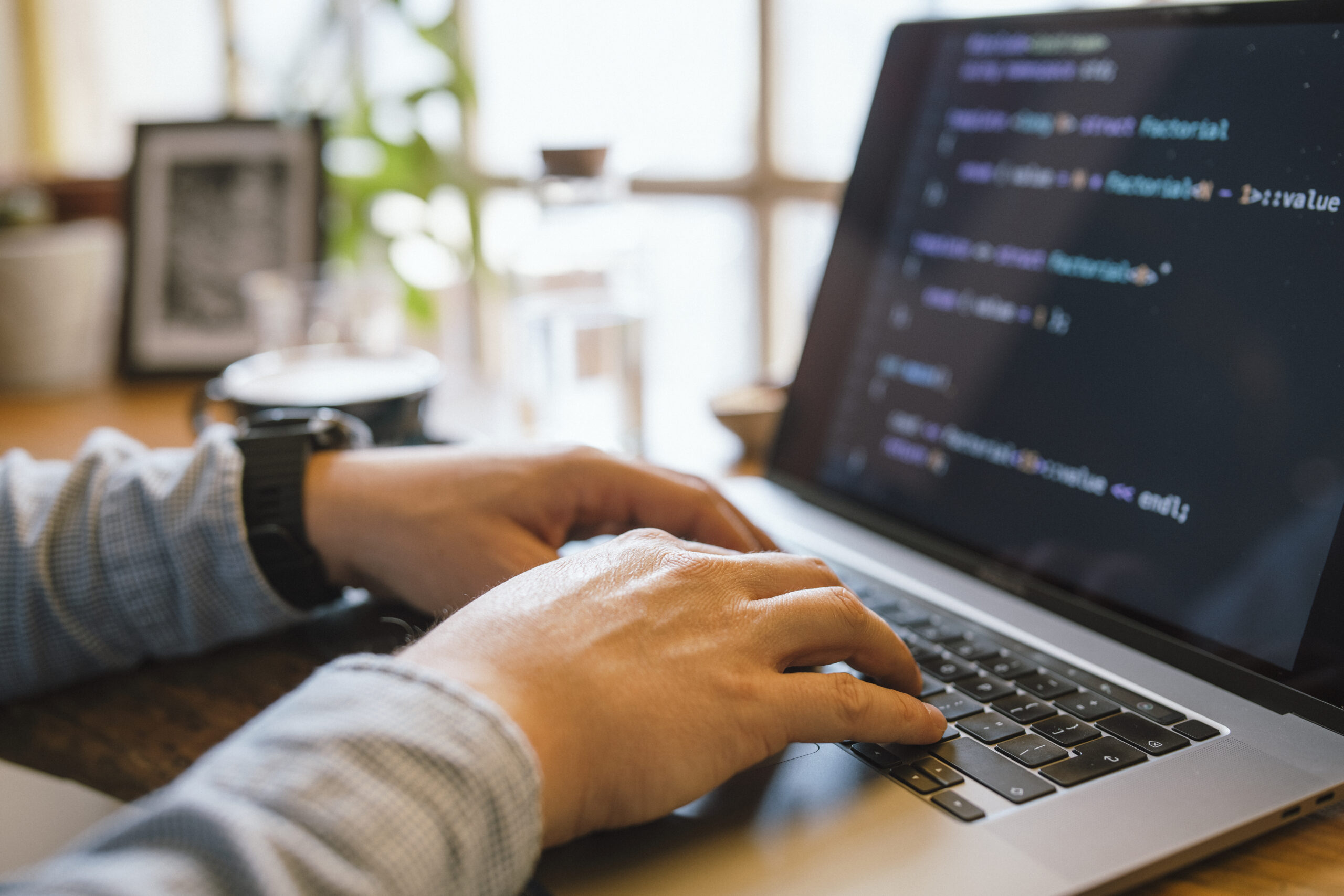
Debugging is Just about the most necessary — yet frequently neglected — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to resolve challenges successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies developers can elevate their debugging expertise is by mastering the tools they use every day. Though producing code is a single A part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Fashionable growth environments arrive Geared up with strong debugging capabilities — but quite a few developers only scratch the area of what these instruments can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, and also modify code on the fly. When applied appropriately, they Permit you to observe accurately how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They enable you to inspect the DOM, keep track of community requests, watch serious-time functionality metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can flip aggravating UI difficulties into workable tasks.
For backend or process-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Manage in excess of operating procedures and memory management. Discovering these equipment can have a steeper Finding out curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, grow to be at ease with version Manage techniques like Git to be familiar with code background, uncover the precise instant bugs were introduced, and isolate problematic adjustments.
Finally, mastering your instruments means going past default options and shortcuts — it’s about establishing an personal familiarity with your enhancement environment in order that when troubles occur, you’re not missing in the dead of night. The greater you already know your applications, the greater time you could shell out resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the challenge
Among the most essential — and sometimes disregarded — actions in efficient debugging is reproducing the issue. Prior to jumping into your code or building guesses, builders want to create a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of prospect, generally resulting in squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What actions brought about the issue? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater detail you may have, the less difficult it gets to be to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered ample information, endeavor to recreate the issue in your neighborhood surroundings. This may suggest inputting the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate producing automated exams that replicate the sting instances or condition transitions associated. These exams not simply help expose the challenge but will also stop regressions Sooner or later.
Sometimes, the issue could possibly be environment-certain — it'd happen only on specific running units, browsers, or below unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a state of mind. It needs endurance, observation, and also a methodical approach. But when you can persistently recreate the bug, you happen to be by now midway to correcting it. With a reproducible situation, You need to use your debugging instruments additional effectively, test prospective fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract grievance into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders need to understand to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, especially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line range? What module or perform brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and Mastering to recognize these can dramatically hasten your debugging process.
Some problems are imprecise or generic, and in People conditions, it’s essential to look at the context wherein the error occurred. Check out linked log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard activities (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal once the method can’t continue.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Center on essential occasions, point out adjustments, enter/output values, and significant determination points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular useful in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify challenges, acquire further visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it is a form of investigation. To efficiently establish and take care of bugs, developers should strategy the method just like a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. Similar to a detective surveys a criminal offense scene, acquire as much pertinent data as it is possible to with no leaping to conclusions. Use logs, examination circumstances, and user reviews to piece with each other a clear photograph of what’s going on.
Upcoming, sort hypotheses. Question on your own: What may very well be resulting in this habits? Have any adjustments not too long ago been produced to the codebase? Has this difficulty happened ahead of beneath comparable circumstances? The intention will be to slim down choices and identify probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a controlled atmosphere. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Pay out shut consideration to little facts. Bugs usually disguise while in the least predicted places—just like a lacking semicolon, an off-by-one particular error, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Non permanent fixes could disguise the true problem, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and aid Some others comprehend your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed challenges in complicated programs.
Generate Tests
Creating exams is among the simplest ways to enhance your debugging expertise and Over-all enhancement efficiency. Tests not just aid catch bugs early but in addition function a security Internet that provides you self esteem when earning changes in your codebase. A effectively-analyzed software is much easier to debug mainly because it helps you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which concentrate on person functions or modules. These little, isolated tests can quickly expose whether a specific bit of logic is Performing as predicted. Every time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Unit exams are especially practical for catching regression bugs—challenges that reappear immediately after Earlier becoming fixed.
Future, combine integration exams and end-to-conclusion exams into your workflow. These assist ensure that many areas of your application do the job jointly easily. They’re significantly valuable for catching bugs that take place in complex devices with several factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your check move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Getting breaks can help you reset your head, cut down frustration, and often see the issue from the new standpoint.
If you're too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable glitches or misreading code you wrote just hrs previously. On this state, your brain results in being fewer successful at challenge-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function during the qualifications.
Breaks Gustavo Woltmann coding also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking oneself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code reviews, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding behaviors moving ahead.
Documenting bugs will also be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. After some time, you’ll begin to see patterns—recurring problems or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts crew performance and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, a lot of the ideal builders will not be the ones who publish perfect code, but people that continuously study from their errors.
In the long run, each bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, much more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.